- Developing with Navigation Controllers and Split Views
- Recipe: Building a Simple Two-Item Menu
- Recipe: Adding a Segmented Control
- Recipe: Navigating Between View Controllers
- Recipe: Presenting a Custom Modal Information View
- Recipe: Page View Controllers
- Recipe: Scrubbing Pages in a Page View Controller
- Recipe: Tab Bars
- Recipe: Remembering Tab State
- Recipe: Building Split View Controllers
- Recipe: Creating Universal Split View - Navigation Apps
- Recipe: Custom Containers and Segues
- One More Thing: Interface Builder and Tab Bar Controllers
- Summary
Recipe: Presenting a Custom Modal Information View
Modal view controllers slide onscreen without being part of your standard view controller stack. Modal views are useful for picking data, updating settings, performing an orthogonal function, or presenting information, tasks that might not match well to your normal hierarchy. Any view controller, including navigation controllers, can present a modal controller as demonstrated in the Chapter 4 walkthroughs. This recipe introduces modal controllers more from code point of view.
Presenting a modal controller branches off from your primary navigation path, introducing a new interface that takes charge until your user explicitly dismisses it. You present a modal controller like this.
[self presentModalViewController:someControllerInstance animated:YES];
The controller that is presented can be any kind of view controller subclass, as well. In the case of a navigation controller, the modal presentation can have its own navigation hierarchy built as a chain of interactions.
Always provide a Done button to allow users to dismiss the controller. The easiest way to accomplish this is to present a navigation controller, adding a bar button to its navigation items. Figure 5-4 shows a modal presentation built around a UIViewController instance using a page curl presentation. You can see the built-in Done button at the top-right of the presentation.
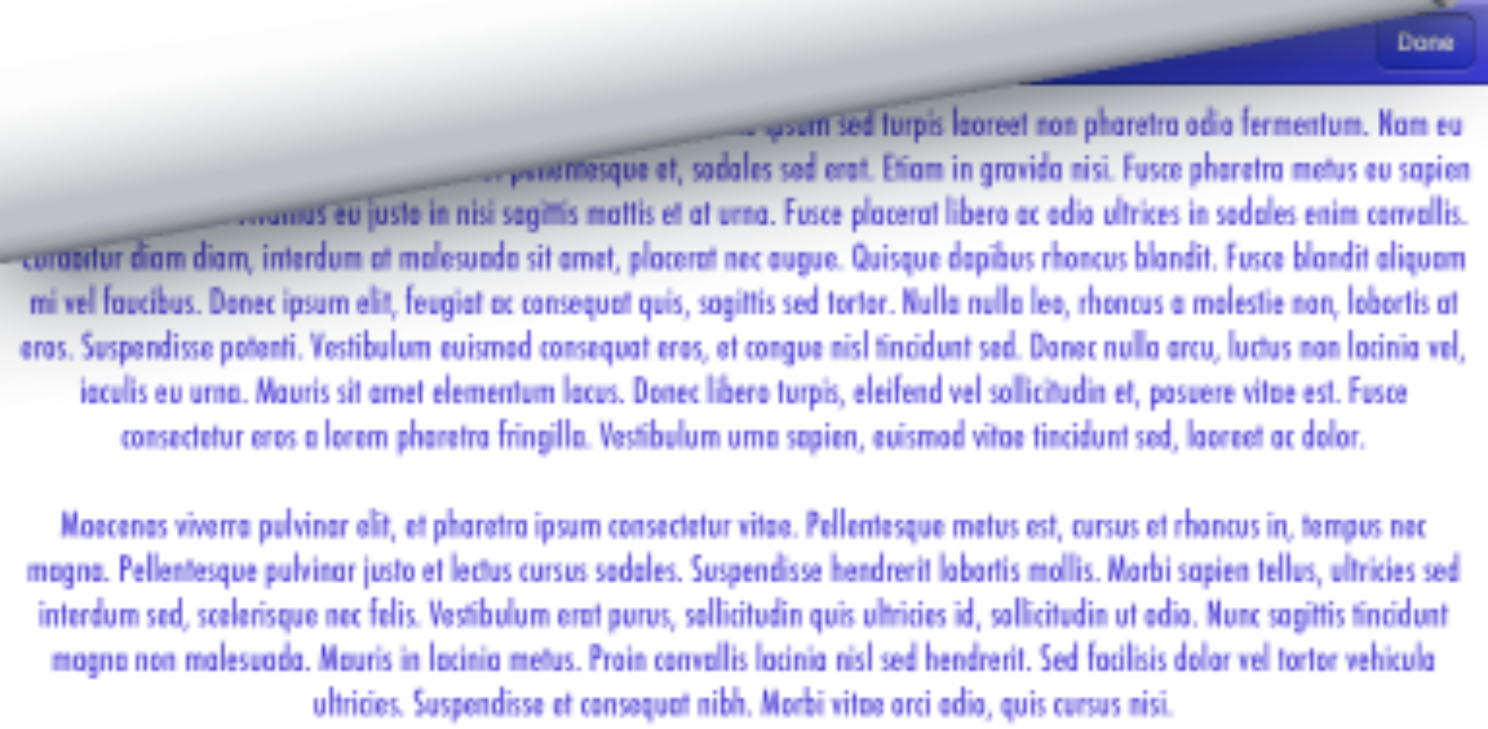
Figure 4 (Click to Enlarge) This modal view is built using UIViewController with a UINavigationBar.
In iOS 5.x, modal presentations can use four transition styles. They are:
- Slide transitions slide a new view over the old.
- Fade dissolves the new view into visibility.
- Flip turns a view over to the "back" of the presentation.
- Curl, which is shown in Figure 5-4, makes the primary view curl up out of the way to reveal the new view beneath it.
In addition to these transition styles, the iPad offers three presentation styles. These include:
- A Full Screen presentation is the default on the iPhone, where the new modal view completely covers both the screen and any existing content. This is the only presentation style that is legal for curls – any other presentation style raises a run-time exception, crashing the application.
- In the Page Sheet, coverage defaults to a portrait aspect ratio, so the modal view controller completely covers the screen in portrait mode and partially covers the screen in landscape mode, as if a portrait-aligned piece of paper were added to the display.
- The Form Sheet display covers a small center portion of the screen, allowing you to shift focus to the modal element while retaining the maximum visibility of the primary application view.
Your modal view controllers must auto rotate. This skeleton demonstrates the simplest possible modal controller you should use. Notice the Interface-Builder-accessible done: method.
@interface ModalViewController : UIViewController - (IBAction)done:(id)sender; @end @implementation ModalViewController - (IBAction)done:(id)sender { [self dismissModalViewControllerAnimated:YES]; } - (BOOL) shouldAutorotateToInterfaceOrientation: (UIInterfaceOrientation)toInterfaceOrientation { return YES; } @end
Storyboards simplify the creation of modal controller elements. Drag in a navigation controller instance, along with its paired view controller, adding a Done button to the provided navigation bar. Set the view controller's class to your custom modal type and connect the Done button to the done: method. Make sure you name your navigation controller in the attributes inspector, so you can use that identifier to load it.
You can either add the modal components to your primary storyboard or create them in a separate file. Recipe 5-4 loads a custom file (Modal~DeviceType.storyboard) but you can just as easily add the elements in your MainStoryboard_DeviceType file.
Recipe 5-4 offers the key pieces for creating modal elements. The presentation is performed in the application's main view controller hierarchy. Here, users select the transition and presentation styles from segmented controls, but these are normally chosen in advance by the developer and set in code or in IB. This recipe offers a toolbox that you can test out on each platform, using each orientation, to explore how each option looks.
Recipe 5-4 Presenting and Dismissing a Modal Controller
// Presenting the controller - (void) action: (id) sender { // Load info controller from storyboard UIStoryboard *sb = [UIStoryboard storyboardWithName: (IS_IPAD ? @"Modal~iPad" : @"Modal~iPhone") bundle:[NSBundle mainBundle]]; UINavigationController *navController = [sb instantiateViewControllerWithIdentfier: @"infoNavigationController"]; // Select the transition style int styleSegment = [(UISegmentedControl *)self.navigationItem.titleView selectedSegmentIndex]; int transitionStyles[4] = { UIModalTransitionStyleCoverVertical, UIModalTransitionStyleCrossDissolve, UIModalTransitionStyleFlipHorizontal, UIModalTransitionStylePartialCurl}; navController.modalTransitionStyle = transitionStyles[styleSegment]; // Select the presentation style for iPad only if (IS_IPAD) { int presentationSegment = [(UISegmentedControl *)[[self.view subviews] lastObject] selectedSegmentIndex]; int presentationStyles[3] = { UIModalPresentationFullScreen, UIModalPresentationPageSheet, UIModalPresentationFormSheet}; if (navController.modalTransitionStyle == UIModalTransitionStylePartialCurl) { // Partial curl with any non-full screen presentation // raises an exception navController.modalPresentationStyle = UIModalPresentationFullScreen; [(UISegmentedControl *)[[self.view subviews] lastObject] setSelectedSegmentIndex:0]; } else navController.modalPresentationStyle = presentationStyles[presentationSegment]; } [self.navigationController presentModalViewController: navController animated:YES]; } - (void) loadView { [super loadView]; self.view.backgroundColor = [UIColor whiteColor]; self.navigationItem.rightBarButtonItem = BARBUTTON(@"Action", @selector(action:)); UISegmentedControl *segmentedControl = [[UISegmentedControl alloc] initWithItems: [@"Slide Fade Flip Curl" componentsSeparatedByString:@" "]]; segmentedControl.segmentedControlStyle = UISegmentedControlStyleBar; self.navigationItem.titleView = segmentedControl; if (IS_IPAD) { NSArray *presentationChoices = [NSArray arrayWithObjects: @"Full Screen", @"Page Sheet", @"Form Sheet", nil]; UISegmentedControl *iPadStyleControl = [[UISegmentedControl alloc] init WithItems:presentationChoices]; iPadStyleControl.segmentedControlStyle = UISegmentedControlStyleBar; iPadStyleControl.autoresizingMask = UIViewAutoresizingFlexibleWidth; iPadStyleControl.center = CGPointMake(CGRectGetMidX(self.view.bounds), 22.0f); [self.view addSubview:iPadStyleControl]; } }