- Application Monitoring
- Setting Up Windows Azure Diagnostics
- Accessing Windows Azure Diagnostic Data
- Windows Azure Diagnostics Configuration Demo
- On-Demand Transfer for the Windows Azure Diagnostics Configuration?
- Modifying the Windows Azure Diagnostics Configuration
- Tradeoffs with Windows Azure Diagnostics Configuration
- Windows Azure Service Dashboard
- Scenarios
- Summary
Windows Azure Diagnostics Configuration Demo
The HelloDiagnostics example shows the basics of how this is done. It consists of a simple web role that has one web page. The page load method writes a simple trace statement indicating that the page is loaded:
protected void Page_Load(object sender, EventArgs e) { System.Diagnostics.Trace.TraceInformation("Page \"" + Request.Url.ToString() + "\" is loaded."); }
The diagnostic configuration is set up in the OnStart method of the web role:
DiagnosticMonitorConfiguration diagnosticConfiguration = DiagnosticMonitor.GetDefaultInitialConfiguration(); diagnosticConfiguration.Directories.ScheduledTransferPeriod = TimeSpan.FromSeconds(2); diagnosticConfiguration.Logs.ScheduledTransferPeriod = TimeSpan.FromSeconds(2); diagnosticConfiguration.Logs.ScheduledTransferLogLevelFilter = LogLevel.Verbose; Microsoft.WindowsAzure.CloudStorageAccount.SetConfigurationSettingPublisher((configName, configSetter) => { configSetter(Microsoft.WindowsAzure.ServiceRuntime.RoleEnvironment GetConfigurationSettingValue(configName)); }); DiagnosticMonitor.Start(diagnosticConnectionString, diagnosticConfiguration);
Most of this code is very straightforward. Since the logs are saved by default, the code just sets the time for the Diagnostic Monitor to transfer them from the filesystem to Azure storage. For traces, we set the transfer to include everything above the verbose level. In a real application you would set longer time spans, but for a demo we want the information available more quickly.
The most mysterious part is the delegate that uses the SetConfigurationSettingPublisher method. Essentially, it specifies where to get the credentials to log into your Azure storage account. We're getting it from the role environment defined in our Azure configuration file.
As with most Azure configuration settings, there's an entry in the definition file and the configuration file. The definition file contains the following entry for diagnostics within the WebRole section:
<Imports> <Import moduleName="Diagnostics" /> </Imports>
The configuration file has the following entries, which specify the actual credentials:
<ConfigurationSettings> <Setting name="Microsoft.WindowsAzure.Plugins.Diagnostics.ConnectionString" value="UseDevelopmentStorage=true" /> <!--<Setting name="Microsoft.WindowsAzure.Plugins.Diagnostics.ConnectionString" value="DefaultEndpointsProtocol=https;AccountName=x;AccountKey=y />--> </ConfigurationSettings>
Our examples run against the local development fabric. If we were to run in the cloud, you would substitute your storage credentials.
The final piece of infrastructure is designed to set up the diagnostic tracing section in the web.config file:
<system.diagnostics> <trace autoflush="true"> <listeners> <add type="Microsoft.WindowsAzure.Diagnostics.DiagnosticMonitorTraceListener, Microsoft.WindowsAzure.Diagnostics, Version=1.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" name="AzureDiagnostics"> <filter type=""/> </add> </listeners> </trace> </system.diagnostics>
Running this application causes two important pieces of diagnostic information to be written to Azure storage: the diagnostic trace information from the web page, and the current configuration of the Diagnostic Monitor. The diagnostic trace information is put in the WADLogs table, and the configuration information is in the blob container wad-control-container.
Video 1 uses the Neudesic Azure Storage Explorer (which can be downloaded from CodePlex) to view the stored information:
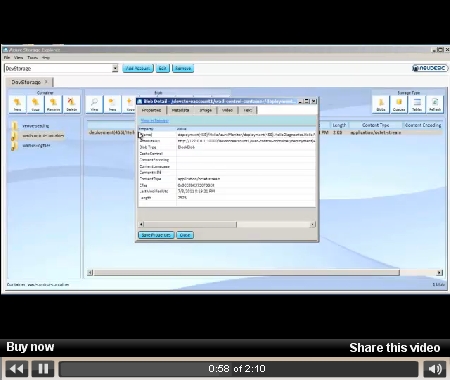
You need to upgrade your Flash Player. You need version 9 or above to view this video. You may download it here. You may also see this message if you have JavaScript turned off. If this is the case, please enable JavaScript and reload the page.
Click here to see full-size video.
If you wanted to keep track of the System and Application event logs, you could add the following lines to the OnStart method:
diagnosticConfiguration.WindowsEventLog.BufferQuotaInMB = 1; diagnosticConfiguration.WindowsEventLog.DataSources.Add("System!*"); diagnosticConfiguration.WindowsEventLog.DataSources.Add("Application!*"); ; diagnosticConfiguration.WindowsEventLog.ScheduledTransferPeriod = TimeSpan.FromSeconds(5); diagnosticConfiguration.WindowsEventLog.ScheduledTransferLogLevelFilter = LogLevel.Verbose;
Of course, you would set the time period between saves to values that make sense for your situation.