- Retrieving and Reading Shared Preferences
- Storing and Updating Shared Preferences
- Creating Preferences Resources Files
- Using the <em>PreferenceActivity</em> Class
Using the PreferenceActivity Class
A PreferenceActivity class (android.preference.PreferenceActivity) is a helper class that will turn your XML preferences resource into a usable screen that behaves much like the Android device settings you may already be familiar with.
To wire up your new preference resource file, begin by creating a new class extending from PreferencesActivity within your application. Override the onCreate() method of your new class to add a few setup method calls. The first call should set the shared preferences name, unless you use the default shared preferences from the PreferenceManager class. To set the name of the shared preferences this activity will manage, call the setSharedPreferencesName() method with the name of the preference group you want to use. Next, tell the activity which preferences resource file to use by calling the addPreferencesFromResource() method with the resource identifier matching the file you just created.
Here is an entire functional class implementation:
public class SampleSettingsActivity extends PreferenceActivity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); PreferenceManager manager = getPreferenceManager(); manager.setSharedPreferencesName("app_prefs"); addPreferencesFromResource(R.xml.preferences); } }
This implementation assumes that you're already using or will use a shared preference group named app_prefs. Figure 1 shows what the preferences screen looks like.
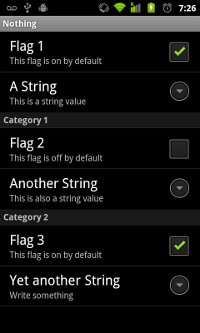
Figure 1 A preferences screen
Figure 2 shows what happens when you click on one of the string preferences.
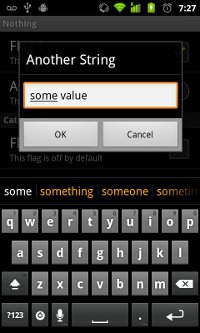
Figure 2 A string preferences entry
By the time the onPause() method of the PreferenceActivity class is reached, the values have been written to the shared preferences file. That is, the preferences values are ready to use right away.
Conclusion
The Android SDK provides many helpful classes for the developer to use to easily handle persistent application preferences, both internally for persistent storage and for consistent user preference management user interfaces. You have learned how to save and retrieve preferences. You also learned how to easily present a settings screen to the user with the same, familiar look-and-feel of the device system settings screens.