The Java Class Library
This book covers how to use the Java language to create your own programs from scratch. You learn all the keywords and operators that form the language and then put them to work writing statements that make a computer do interesting and useful things.
Although this approach is the best way to learn Java, it’s a bit like showing someone how to build a car by making her build every part of the car from scratch first.
A lot of work already is done for you as a Java programmer, provided you know where to look for it.
Java comes with an enormous collection of code you can utilize in your own programs called the Java Class Library. This library is a collection of thousands of classes, many of which can be used in the programs that you write.
The classes can be put to work in your programs in a manner somewhat similar to using a variable.
A class is used to create an object, which is like a variable but far more sophisticated. An object can hold data, like a variable does, and also perform tasks, like a program.
Oracle offers comprehensive documentation for the Java Class Library on the Web at http://download.java.net/jdk9/docs/api. This page is shown in Figure 4.3.
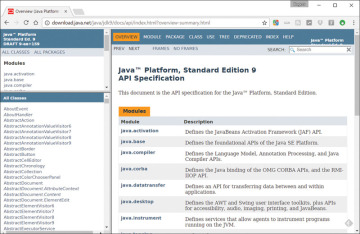
FIGURE 4.3 The Java Class Library documentation.
Java classes are organized into packages, which serve a similar function to a file folder on a computer. The programs you have created thus far belong to the com.java24hours package.
The home page for the documentation is divided into frames. The largest frame lists all of the packages that compose the Java Class Library, along with a description of each one.
The names of the packages help describe their purpose. For instance, java.io is a set of classes for input and output from disk drives, Internet servers, and other data sources; java.time contains classes related to times and dates; and java.util collects helpful utility classes.
On the documentation home page, in the largest frame is a list of packages with a short description of each one. Click the name of a package to learn more about it. A page loads listing the classes in the package.
Each class in the Java Class Library has its own page of documentation on this reference site, which consists of more than 22,000 pages. (You don’t have to read all of them now, or ever.)
For this hour’s final project, you will poke around the library and use an existing Java class to do some work for you.
The Dice program uses the Random class in the java.util package, which can be used to create random numbers.
The first thing you do in the program to use this class is import its package with this statement:
import java.util.*;
This makes it possible to refer to the Random class without using its full name, which is java.util.Random. Instead, you can simply refer to it as Random. The asterisk makes it possible to refer to all classes in a package by their shorter names.
If you only wanted to import the Random class, this statement would be used:
import java.util.Random;
A Java program can be thought of as an object that performs a task (or tasks). The Random class is a template that can be used to create a Random object. To create an object, use the new keyword followed by the name of the class and parentheses:
Random generator = new Random();
This creates a variable named generator that holds a new Random object. The object is a random-number generator that can produce random numbers. The tasks an object can do are called methods.
For this program, the object’s nextInt() method will be employed to produce a random integer value:
int value = generator.nextInt();
Integers in Java range from −2,147,483,648 to 2,147,483,647. The generator chooses one of these numbers at random and it is assigned to the value variable.
Without the Random class from the Java Class Library, you’d have to create your own program to produce random numbers, which is a highly complex task. Random numbers are useful in games, educational programs, and other programs that must do something randomly.
In NetBeans, create a new empty Java file, name it Dice, and put it in the package com.java24hours. When the source code editor opens, enter the text of Listing 4.3 into that window and then click the Save button (or choose the menu command File, Save).
LISTING 4.3 The Full Text of Dice.java
1: package com.java24hours; 2: 3: import java.util.*; 4: 5: class Dice { 6: public static void main(String[] arguments) { 7: Random generator = new Random(); 8: int value = generator.nextInt(); 9: System.out.println("The random number is " 10: + value); 11: } 12: }
Run the program by choosing Run, Run File. The output is shown in Figure 4.4, although your number will be different. (Actually, there’s a one in four billion chance it will be the same number, which is even worse odds than a lottery.)
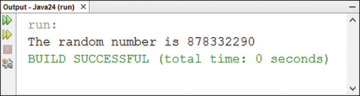
FIGURE 4.4 The output of the Dice program.
You now have a randomly selected integer to call your own. Take good care of it.
The Random class, like all classes in the Java Class Library, has extensive documentation you can read on Oracle’s site. It describes the purpose of the class, the package it belongs to, how to create an object of this class, and what methods it has that can be called to make it do something.
Follow these steps to see this documentation:
In your web browser, load the page http://download.java.net/jdk9/docs/api.
In a navigation bar atop the page at upper left, click the Frames link. The documentation opens in several frames.
In the Modules frame at upper left, click java.base.
In the main frame, scroll down until you see the link to the package java.util. Click this link. Documentation for the package is displayed.
In the main frame, scroll down and click the Random link.
As a Java programmer with slightly under four hours’ experience, you likely will find the documentation to be mind-bendingly tough to understand. This is no cause for alarm. It’s written for experienced programmers.
But as you read this book and become curious about how Java’s built-in classes are being used, you can get some value from looking at the official documentation for a class. One way to use it is to look up the methods in that class, each of which performs a job.
On the Random documentation, you can scroll down to the explanation for the nextInt() method, which was used on line 8 of Listing 4.3. Figure 4.5 shows this section of the page.
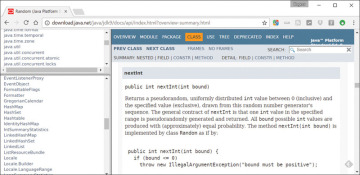
FIGURE 4.5 Oracle’s documentation for the Random class.